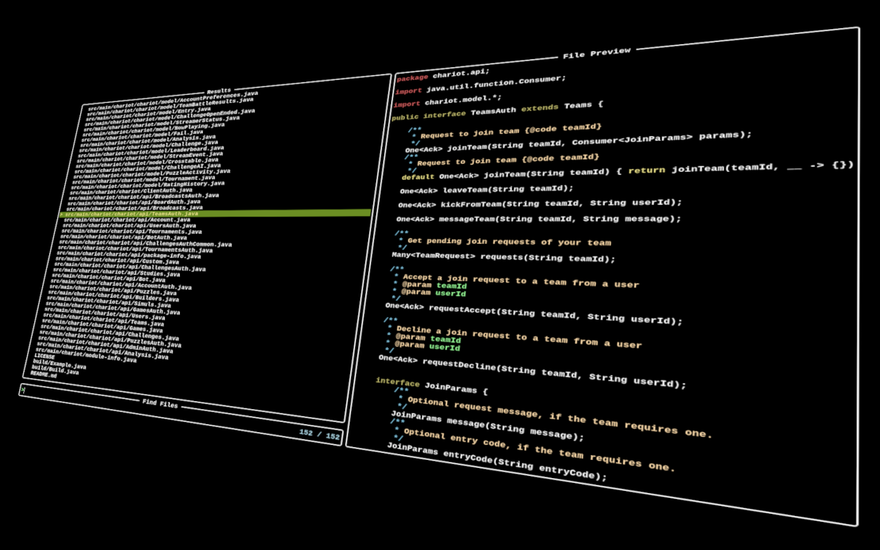
Chariot - Java client for the Lichess API
In addition to Lichess's visible features - there is also an APILichess has a lot of features and is constantly getting more!
In addition to the visible features, there also exists an API (Application Programming Interface) which can be used by developers to write applications which can interact with Lichess - such as Bots and OpeningTree.com.
The API is accessed by using a set of documented HTTP operations and this post is about a Java library, Chariot, which implements this Lichess API - making these operations easily available for Java applications.
Here's how the code could look for an application which fetches a Lichess Team and outputs how many members it has:
import chariot.Client;
import chariot.model.*;
void main() {
// Create a client
var client = Client.basic();
// Lichess Swiss
// https://lichess.org/team/lichess-swiss
String teamId = "lichess-swiss";
// Fetch info about the team from Lichess
One<Team> teamResult =
client.teams().byTeamId(teamId);
// Create a message from the result
String message = switch(teamResult) {
case Entry(var team) ->
STR."\{team.nbMembers()} members in \{team.name()}";
case Fail(int status, _) ->
STR."Failure - Status code \{status}";
case None() -> "<empty>";
};
// One could also ignore type checking,
// and just assume it was successful,
// but the .get() could throw exception
// if result wasn't successful - like:
Team team = teamResult.get();
String teamName = team.name();
// Show the message
System.out.println(message);
// Prepare a stream of the Lichess Swiss members from Lichess
Many<TeamMember> memberResult =
client.teams().usersByTeamId(teamId);
// Only receive the first result (if any),
// which is the newest member,
// show the member name
memberResult.stream()
.findFirst()
.ifPresent(newest -> System.out.println(
STR."Newest member: \{newest.user().name()}"));
}
A listing of all methods in Chariot can be found here
Edit, the previous example was written for Java 17 -but I've updated it for Java 21 to make use of String Templates and Record Patterns -so to run the above example, one needs to use at least Java 21 (with preview features enabled).Note, it is still possible to use Chariot with Java 17 - but newer versions are ok too.
Latest Java can be downloaded and upacked from here, and one can use a published version of Chariot from Maven Central (download jar into a directory) and then Copy/Paste the above code into a file named Team.java
And then running it:
$ java --enable-preview --source 21 -cp chariot-0.0.78.jar Team.java
422087 members in Lichess Swiss
Newest member: charleton_orlando
or with paths to the files:
$ C:\path\to\downloaded\jdk-21\bin\java --enable-preview --source 21 -cp C:\path\to\downloaded\chariot-0.0.78.jar C:\path\to\downloaded\Team.java
422087 members in Lichess Swiss
Newest member: charleton_orlando
There's also a Maven example project available, which can be handy since many IDEs such as IntelliJ IDEA and VS Code have support for opening Maven projects and getting the documentation, code completion and all the Good Stuff in place out-of-the-box.
This is an example application, Team Check, which can be used to visualize members of Lichess teams (in a somewhat silly way...)
It may be a silly application, but at least its code serves to demo the following features:
- Searching for Lichess teams
- List members of Lichess teams
- Login/Logout, if one wants to access authorized parts of the API
- Kick members from a Lichess team (if you are the team leader)
In case you don't like Java, people have also written API clients in other languages, such as Python for instance - like this one named Berserk.
And it is of course also possible to use the HTTP operations directly, without
using a library.
Should you choose to make a Lichess application, using any method,
consider blogging about it!
That's it for now,
thank you for your time! :)